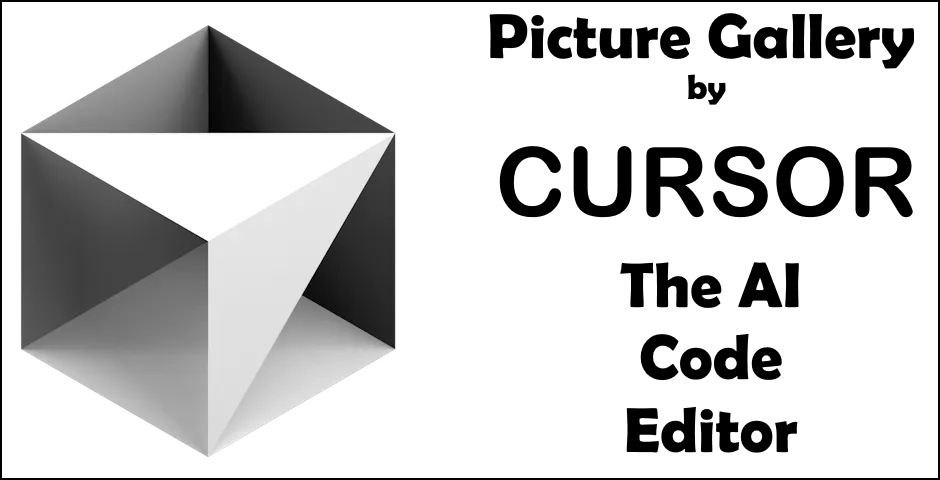
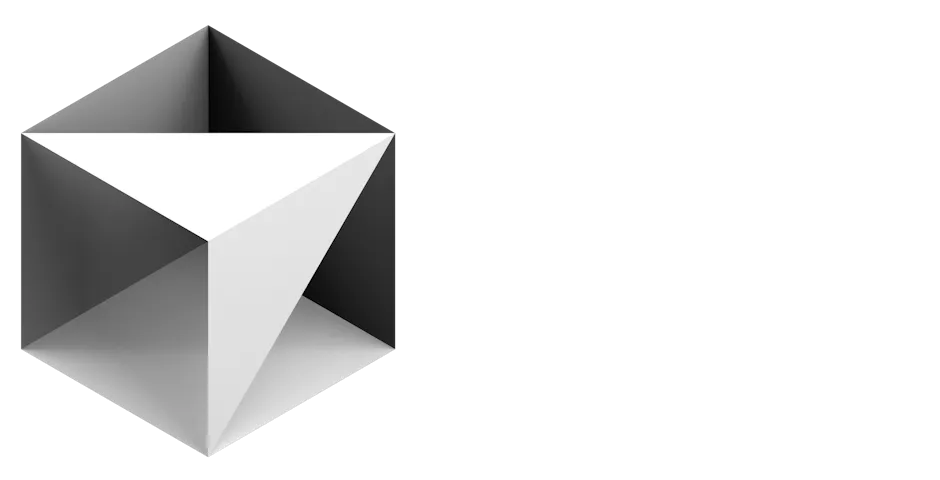
Using Cursor to create an Image Gallery with Astro and Vue
I used Cursor to build the Pets image gallery on this site and then told it to write a blog post about it, this is what it wrote:
Building a performant image gallery that works well across all devices while maintaining good user experience can be challenging. In this post, I’ll walk through how I built my pet gallery using Astro and Vue, focusing on performance, user experience, and maintainability.
Key Features
- Responsive Masonry Layout: Fluid grid that adapts to any screen size
- Smart Image Loading:
- Lazy loading for better performance
- Progressive loading with mobile-first thumbnails
- Automatic image optimization
- Advanced Metadata:
- EXIF data extraction for dates
- Automatic dimension calculation
- Intelligent fallbacks
- Rich User Experience:
- Mobile-friendly touch controls
- Keyboard navigation in modal view
- Real-time search filtering
- Performance Optimizations:
- WebP format with quality presets
- Responsive image srcsets
- Layout shift prevention
Here’s the core interface we’ll be working with:
interface Pet {
id: number;
name: string;
image: string;
thumbnail: string;
mobileThumbnail: string;
description?: string;
dateTaken?: string | null;
dimensions: {
original: { width: number; height: number };
thumbnail: { width: number; height: number };
mobileThumbnail: { width: number; height: number };
};
}
The Architecture
The gallery consists of two main components:
- An Astro page (
pets.astro
) that handles image processing and data preparation - A Vue component (
PetGallery.vue
) that manages the UI and interactions
This separation allows us to leverage Astro’s server-side capabilities for heavy lifting while maintaining a smooth client-side experience with Vue.
Image Processing with Astro
Reading Images from the File System
In pets.astro
, we start by reading images from a directory:
const petFiles = await Astro.glob('/public/img/pets/*.{jpg,JPG}');
Extracting EXIF Data
One of the most interesting aspects is extracting EXIF data from images. This gives us information like when the photo was taken:
async function getExifData(filePath: string): Promise<ExifData | null> {
try {
const buffer = await fs.readFile(filePath);
// Find the EXIF marker (0xFF 0xE1)
let offset = 0;
while (offset < buffer.length - 1) {
if (buffer[offset] === 0xFF && buffer[offset + 1] === 0xE1) {
const exifLength = buffer.readUInt16BE(offset + 2);
const exifData = buffer.slice(offset + 4, offset + 2 + exifLength);
try {
const parsedExif = exif(exifData) as ExifData;
return parsedExif;
} catch (parseError) {
console.error('Error parsing EXIF data:', parseError);
return null;
}
}
offset++;
}
return null;
} catch (error) {
console.error(`Error reading file ${filePath}:`, error);
return null;
}
}
Image Dimensions and Thumbnails
Getting accurate image dimensions is crucial for preventing layout shifts:
async function getImageDimensions(filePath: string) {
try {
const metadata = await sharp(filePath).metadata();
return {
width: metadata.width || 0,
height: metadata.height || 0
};
} catch (error) {
console.error(`Error getting dimensions for ${filePath}:`, error);
return { width: 0, height: 0 };
}
}
We calculate thumbnail dimensions while maintaining aspect ratio:
function calculateThumbnailDimensions(
originalWidth: number,
originalHeight: number,
targetWidth: number
) {
const aspectRatio = originalWidth / originalHeight;
const thumbnailHeight = Math.round(targetWidth / aspectRatio);
return {
width: targetWidth,
height: thumbnailHeight
};
}
The Vue Gallery Component
Responsive Image Loading
The gallery uses different image sizes for different devices:
<template>
<img
:src="pet.mobileThumbnail"
:srcset="`${pet.mobileThumbnail} 150w, ${pet.thumbnail} 300w`"
:sizes="'(max-width: 640px) 150px, 300px'"
:alt="pet.name"
:width="pet.dimensions.thumbnail.width"
:height="pet.dimensions.thumbnail.height"
:style="`aspect-ratio: ${pet.dimensions.original.width} / ${pet.dimensions.original.height}`"
loading="lazy"
class="w-full h-auto block rounded-lg"
>
</template>
Lazy Loading Implementation
We use the Intersection Observer API to load images only when they’re about to enter the viewport:
const setupIntersectionObserver = () => {
const observer = new IntersectionObserver(
(entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const petId = Number(entry.target.getAttribute('data-pet-id'))
if (petId) {
loadedImages.value.add(petId)
}
observer.unobserve(entry.target)
}
})
},
{
rootMargin: '50px 0px',
threshold: 0.1
}
)
setTimeout(() => {
document.querySelectorAll('[data-pet-id]').forEach(el => {
observer.observe(el)
})
}, 100)
}
Search Functionality
The gallery includes real-time search with regex support:
const filteredPets = computed(() => {
if (!searchQuery.value) return randomizedPets.value;
const query = searchQuery.value.toLowerCase();
try {
const regex = new RegExp(query, 'i');
return randomizedPets.value.filter(pet =>
regex.test(pet.name) ||
(pet.description && regex.test(pet.description))
);
} catch (e) {
// Fallback to simple includes if regex is invalid
return randomizedPets.value.filter(pet =>
pet.name.toLowerCase().includes(query) ||
(pet.description && pet.description.toLowerCase().includes(query))
);
}
})
Modal View with Touch Support
The modal view includes touch support for mobile devices:
const handleTouchStart = (e: TouchEvent) => {
touchStartX.value = e.touches[0].clientX
}
const handleTouchMove = (e: TouchEvent) => {
touchEndX.value = e.touches[0].clientX
}
const handleTouchEnd = () => {
const swipeDistance = touchEndX.value - touchStartX.value
if (Math.abs(swipeDistance) > 50) {
if (swipeDistance > 0) {
navigatePet('prev')
} else {
navigatePet('next')
}
}
}
Performance Considerations
Layout Shift Prevention
- Pre-calculating and setting image dimensions
- Using aspect-ratio CSS
- Placeholder loading states
Load Time Optimization
- Lazy loading images
- Progressive image loading (mobile thumbnails → desktop thumbnails → full size)
- Efficient image formats (WebP with JPEG fallback)
Memory Management
- Cleaning up observers
- Managing modal state
- Efficient event listeners
Accessibility Features
Keyboard Navigation
- Arrow keys for image navigation
- Escape key to close modal
- Focus management for modal
Screen Reader Support
- Proper alt text for images
- ARIA labels and roles
- Semantic HTML structure
Touch Interactions
- Swipe gestures for mobile
- Touch-friendly hit areas
- Responsive controls
Conclusion
Building a performant image gallery requires careful consideration of many factors, from initial image processing to final user interactions. By leveraging Astro’s server-side capabilities and Vue’s reactive system, we’ve created a gallery that’s both performant and user-friendly.
The complete code for this gallery is available in the repository, and you can see it in action on my pets page.
Example Implementation
Here’s a small example of the gallery in action:
<PetGallery
:pets="[
{
id: 1,
name: 'Zeus',
image: '/img/pets/Zeus Head Tilt 1.jpg',
thumbnail: '/img/pets/Zeus Head Tilt 1.jpg?w=300',
mobileThumbnail: '/img/pets/Zeus Head Tilt 1.jpg?w=150',
dimensions: {
original: { width: 1200, height: 800 },
thumbnail: { width: 300, height: 200 },
mobileThumbnail: { width: 150, height: 100 }
}
},
// ... more examples
]"
/>
Stay tuned for more posts about web development and performance optimization!